Gary Rosenberry, Senior Software Developer-Newmoyer Geospatial Solutions
Gary is an experienced Software Engineer with a demonstrated history of working in the computer software industry. Skilled in GO, Java, JavaScript, Spring, React/Redux, SQL, Government, Management. Strong engineering professional with a Bachelor’s degree focused in Computer Science and Programming from Shippensburg University of Pennsylvania.
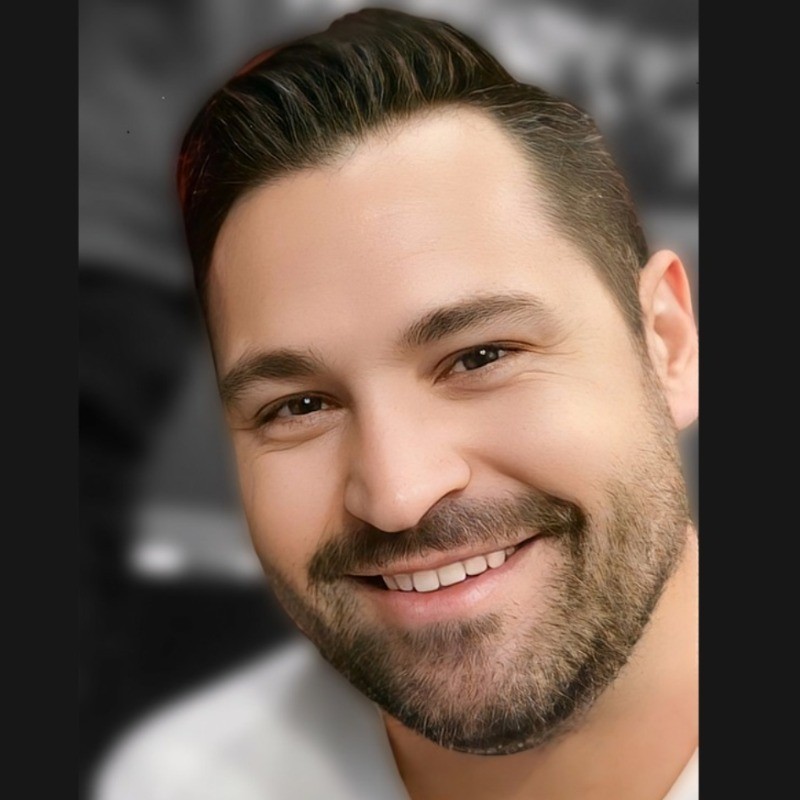
Introduction
As a full-stack developer since November 2016, I’ve had the opportunity to work on a variety of custom web application solutions across different tech stacks. In recent years, I’ve shifted my focus toward leading front-end development efforts, primarily working with React. My experience spans Java, Go, and JavaScript, but my main expertise lies in building and maintaining React-based projects.
For the past four years, Redux has been my go-to tool for managing and organizing application state, and more recently, Redux Toolkit has become an essential part of my workflow. One of the most overlooked yet crucial aspects of working with these technologies is establishing a scalable and maintainable folder structure. A well-organized project can make a significant difference in code maintainability, collaboration, and long-term scalability.
In this article, I’ll share an example of structuring a React application using Redux Toolkit effectively. While this is not the only way to organize a project, it provides a solid foundation that can be adapted to different needs. Whether you’re starting a new project or refactoring an existing one, having a structured approach will save you and your team countless hours down the line.
Why Folder Structure Matters
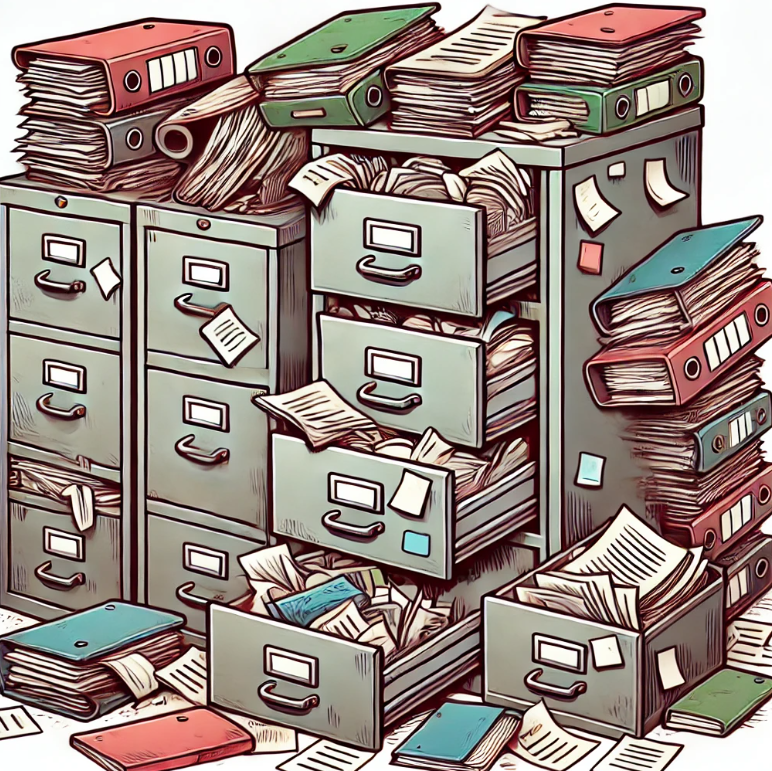
The Downside of Poor Organization
A poorly designed or inconsistent folder structure leads to wasted time and unnecessary confusion. Without clear guidelines, developers might struggle to find files, leading to delays and inefficiencies. More importantly, a lack of organization can make it difficult to understand how different parts of the application interact, increasing the risk of introducing bugs and technical debt.
The Benefits of a Well-Organized Project
Core Principles of a Good Folder Structure
A well-structured project should be logical, scalable, and easy to navigate. Here are the key principles to follow:
src/
app/
App.tsx
store.ts
features/
auth/
components/
AuthForm.tsx
authSlice.ts
dashboard/
components/
Dashboard.tsx
dashboardSlice.ts
Feature-Based Organization
Instead of organizing files solely by type (e.g., a global components/ or redux/ folder), structure them around distinct features.
This method ensures that each feature is self-contained, making it easier to update or remove functionality without affecting unrelated parts of the application.
API Folder with RTK Query
To manage API interactions efficiently, we separate API logic into its own folder. This is a personal choice, but I prefer abstracting my API into its own folder. This allows me to code-split the API and re-use these pieces across features.
src/
api/
api.ts
authApi.ts
dashboardApi.ts
Base API Setup (api. ts)
import { createApi, fetchBaseQuery } from '@reduxjs/toolkit/query/react';
export const api = createApi({
reducerPath: 'api',
baseQuery: fetchBaseQuery({ baseUrl: '/api' }),
endpoints: () => ({}), // Additional endpoints are injected separately
});
Injecting Feature-Specific Endpoints (authApi. ts)
import { api } from './api';
export const authApi = api.injectEndpoints({
endpoints: (builder) => ({
login: builder.mutation({
query: (credentials) => ({
url: '/auth/login',
method: 'POST',
body: credentials,
}),
}),
}),
});
export const { useLoginMutation } = authApi;
This approach allows us to add API endpoints dynamically, keeping the code modular and easier to manage.
Common Folder for Shared Logic
To avoid redundant code, we place reusable logic inside a common/ directory.
src/
common/
hooks/
useAuth.ts
useDebounce.ts
utils/
dateFormatter.ts
apiHelper.ts
components/
Button.tsx
Modal.tsx
Hooks (hooks/)
import { useGetUserQuery } from '../api/authApi';
export const useAuth = () => {
const { data: user, isLoading } = useGetUserQuery();
return { user, isLoading, isAuthenticated: !!user };
};
Utility Functions (utils/)
export const formatDate = (date: string) => {
return new Date(date).toLocaleDateString();
};
Reusable Components (components/)
export const Button: React.FC<{ text: string; onClick: () => void }> = ({ text, onClick }) => {
return <button className="px-4 py-2 bg-blue-500 text-white rounded" onClick={onClick}>{text}</button>;
};
Caution When Editing common/
Since shared logic affects multiple parts of the application, updates should be handled carefully:
Final Thoughts
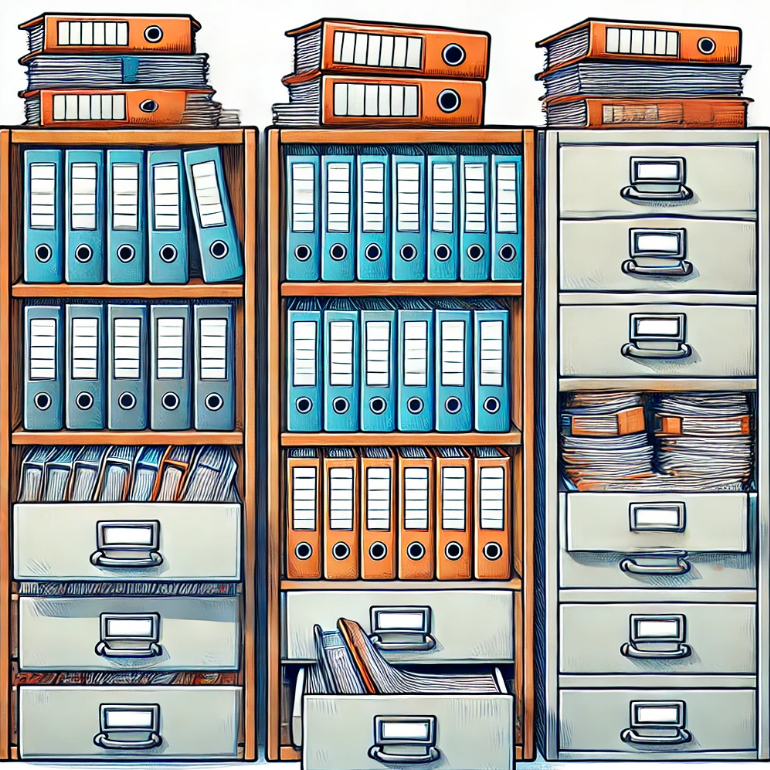
A good folder structure isn’t about following rigid rules—it’s about creating a setup that makes your project scalable, maintainable, and easy to navigate. While this isn’t the only way to structure a React project, it provides a strong foundation that can be adapted to different team preferences and project requirements. By adopting a feature-based organization, a modular API layer, and a structured common folder, you can reduce technical debt, improve collaboration, and boost development efficiency.